Cybersecurity Awareness Month: Fortifying Web Development with React and Next.js
October ushers in Cybersecurity Awareness Month, a time for us, the architects of the digital world, to reflect on the security of the applications we build. As web developers, our choices in tools and frameworks play a pivotal role in defending against modern threats. This month, let's delve into how React and Next.js not only empower us to create dynamic web applications but also offer robust security features.
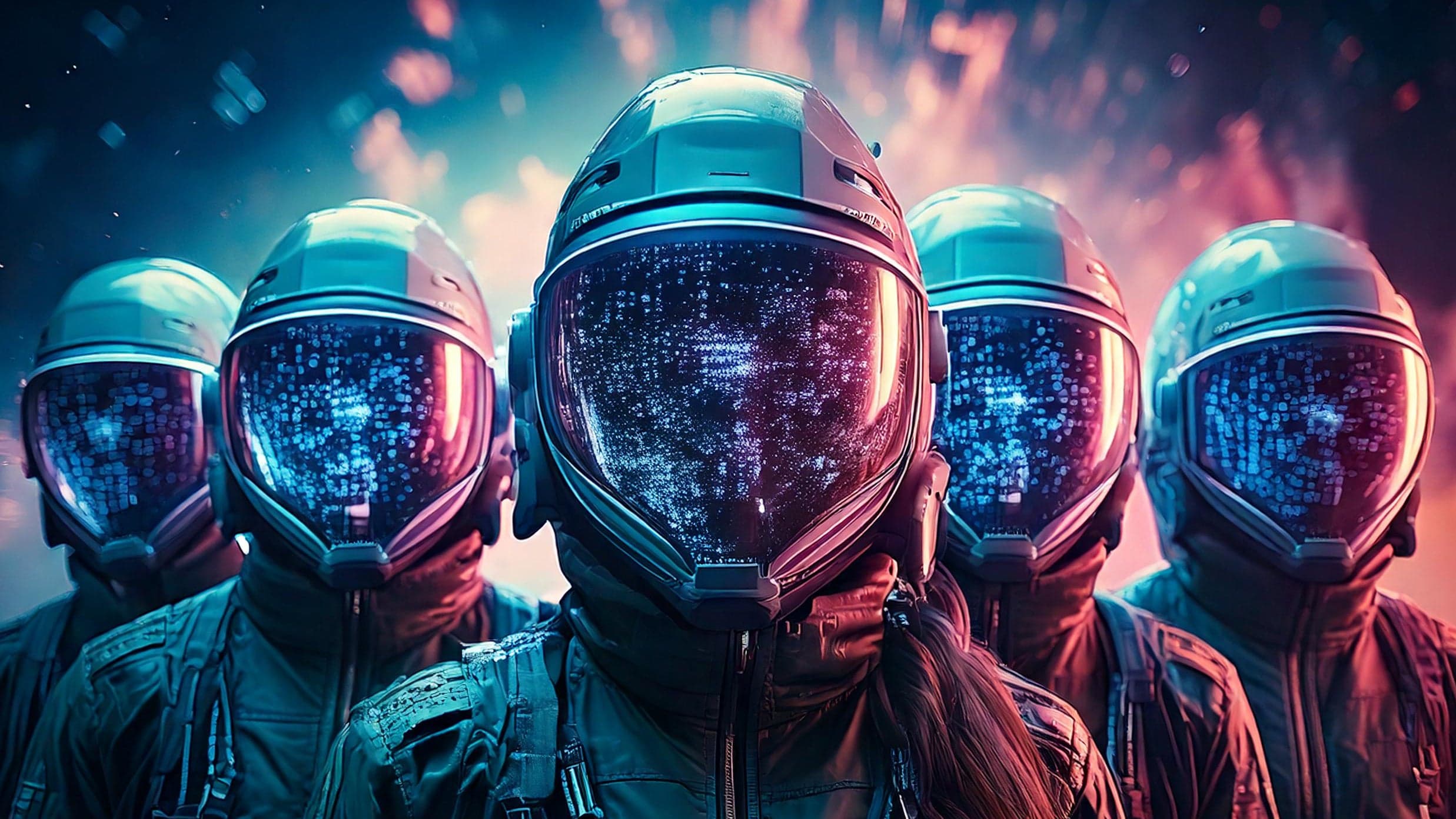
The Evolving Threat Landscape
The cybersecurity landscape is in constant flux, with attackers exploiting new vulnerabilities and techniques. Supply chain attacks, open-source vulnerabilities, and API breaches have underscored the need for heightened security awareness in web development. While specific incidents from 2024 are beyond our current knowledge, the patterns of past events guide us in fortifying our applications.
Supply chain attacks target the dependencies and packages that our applications rely on. Malicious code injected into popular libraries can compromise thousands of applications downstream. Open-source vulnerabilities, such as those found in widely-used NPM packages, highlight the risks inherent in leveraging third-party code. API breaches, resulting from improper authentication and data handling, expose sensitive user information and erode trust.
Why React and Next.js?
React and Next.js have become staples in modern web development, not just for their performance and developer experience but also for their inherent security advantages.
Component-Based Architecture
React's component-based architecture promotes encapsulation. Each component manages its own state and rendering logic, reducing the risk of unintended side effects that can lead to security vulnerabilities.
Server-Side Rendering with Next.js
Next.js enhances React by enabling server-side rendering (SSR), which can improve security by reducing the exposure of sensitive data in the client-side code. SSR can also help mitigate certain injection attacks by pre-rendering content on the server.
Built-In Security Features
Both React and Next.js come with features that help prevent common web vulnerabilities:
- Automatic Output Encoding: React escapes values embedded in JSX by default, protecting against Cross-Site Scripting (XSS) attacks.
- Strict Mode: React's Strict Mode helps identify unsafe lifecycles and legacy API usage.
- Static Site Generation (SSG): Next.js supports SSG, which can reduce the attack surface by serving pre-generated static pages.
Strengthening Our Defenses with React and Next.js
Preventing XSS Attacks
React's default behavior of escaping content helps prevent XSS attacks. However, using dangerouslySetInnerHTML can introduce vulnerabilities if not handled correctly.
Vulnerable Code:
If content includes malicious scripts, they will be executed in the user's browser. To mitigate this, always sanitize any HTML content before rendering.
Secure Code with Sanitization:
By sanitizing content with a library like DOMPurify, we remove any malicious scripts before rendering.
Protecting Against CSRF Attacks
Cross-Site Request Forgery (CSRF) attacks trick users into submitting unauthorized requests. Next.js can leverage built-in APIs and middleware to implement CSRF protection.
Implementing CSRF Tokens:
By integrating CSRF tokens in API routes, we ensure that only legitimate requests are processed.
Secure Authentication with NextAuth.js
Authentication is a critical component of web security. Next.js integrates seamlessly with NextAuth.js to provide secure authentication mechanisms.
Setting Up NextAuth.js:
By configuring NextAuth.js with JWT encryption, we enhance the security of user sessions.
Implementing Content Security Policy (CSP)
Next.js allows custom server configuration to set security headers like CSP, which controls resources the client is allowed to load.
Adding Security Headers:
Setting a strict CSP reduces the risk of XSS attacks by only allowing scripts from trusted sources.
Regularly Updating Dependencies
Using tools to audit and update dependencies is crucial. For React and Next.js projects, npm audit
can identify vulnerabilities.
Auditing Dependencies:
Automating this process in CI/CD pipelines ensures that vulnerabilities are addressed promptly.
Automating Dependency Updates with Dependabot
Keeping dependencies up to date is a critical aspect of maintaining application security. GitHub's Dependabot automates this process by monitoring your project's dependencies and automatically generating pull requests when updates are available, especially for security vulnerabilities.
Setting Up Dependabot:
Enable Dependabot in Your Repository: Dependabot is integrated into GitHub, so you can enable it directly from your repository settings.
Configure Dependabot via dependabot.yml
: Create a .github/dependabot.yml
file in your repository to customize Dependabot's behavior.
package-ecosystem
: Specifies the package manager, e.g.,npm
for Node.js projects.directory
: The directory where thepackage.json
file is located.schedule
: Defines how often Dependabot checks for updates.open-pull-requests-limit
: Limits the number of open pull requests.
Review and Merge Dependabot Pull Requests: Dependabot will create pull requests for dependency updates. Review the changes, run your tests, and merge when ready.
Benefits of Using Dependabot:
- Proactive Security: Automatically receive updates for dependencies with known vulnerabilities.
- Continuous Updates: Stay on the latest versions of dependencies, reducing the risk of compatibility issues.
- Integration with CI/CD: Dependabot pull requests can trigger your CI/CD pipeline, ensuring that updates are tested before merging.
Example of a Dependabot Pull Request:
Dependabot's pull requests include detailed information about the update:
- Changelog and Release Notes: Provides context on what has changed.
- Impact Analysis: Highlights potential breaking changes or security fixes.
- Compatibility Score: Estimates the likelihood of a successful upgrade.
Automated Security Updates:
Dependabot can be configured to automatically merge minor updates or patches that pass your tests, streamlining the update process.
Embracing TypeScript for Type Safety
TypeScript adds a layer of type safety to JavaScript, helping to catch errors at compile time that could lead to runtime vulnerabilities.
Using TypeScript with Next.js:
TypeScript can prevent issues like undefined variables or mismatched types, which can be exploited in certain attack vectors.
Adopting a Security-First Mindset
Beyond the tools and frameworks, adopting a security-first mindset is essential.
Code Reviews and Pair Programming
Regular code reviews and pair programming sessions can catch potential security issues early. Collaborative practices encourage knowledge sharing and collective responsibility.
Continuous Integration and Deployment (CI/CD)
Integrate security checks into your CI/CD pipeline. Tools like GitHub Actions can run tests and audits automatically.
Example GitHub Action for Security Scanning:
Staying Informed
Keep abreast of the latest security trends and vulnerabilities. Subscribe to security advisories related to React, Next.js, and their ecosystems.
Looking Ahead: Our Role in Cybersecurity
As developers, we are guardians of the applications we build. The choices we make—from the frameworks we select to the code we write—have profound implications for security.
Take this month to:
- Audit Your Projects: Review your React and Next.js applications for potential security vulnerabilities.
- Enhance Your Knowledge: Explore security features and best practices within these frameworks.
- Engage with the Community: Share your experiences and learn from others in developer forums and at local meetups.